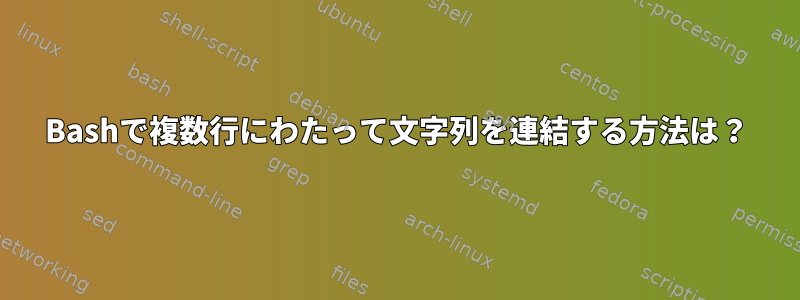
script.shから:
#!/bin/bash
# Above is a while statement, so the printf has a indent(actually are 3 spaces)
printf "I am a too long sentence in line1, I need to switch to a new line. \n\
I'd like to be at the head of this line2, but actually there are 3 redundant spaces \n"
コードで述べたように、次のように表示されます。
I am a too long sentence in line1, I need to switch to a new line.
I'd like to be at the head of this line2, but actually there are 3 redundant spaces
printf
この問題を解決するには、すべての行にaを使用する必要があります。良い:
printf "I am a too long sentence in line1, I need to switch to a new line. \n"
printf "I'd like to be at the head of this line2, but actually there are 3 redundant spaces \n"
あまりにも愚かなようですが、なぜ以下のように文字列を連結するのですか?
printf {"a\n"
"b"}
# result should be like below
a
b
答え1
printf
一般的な方法を使用できます。最初の文字列は型を定義し、次の文字列はパラメータです。
例:
#!/bin/bash
printf '%s\n' "I am a too long sentence in line1, I need to switch to a new line."\
"I'd like to be at the head of this line2, but actually there are 3 redundant spaces."\
"I don't care how much indentation is used"\
"on the next line."
出力:
I am a too long sentence in line1, I need to switch to a new line.
I'd like to be at the head of this line2, but actually there are 3 redundant spaces.
I don't care how much indentation is used
on the next line.
答え2
@Freddyの方法はprinfに基づいています。インデントなしで複数行の文字列を作成する一般的な方法を見つけました。
#!/bin/bash
concat() {
local tmp_arg
for each_arg in $@
do
tmp_arg=${tmp_arg}${each_arg}
done
echo ${tmp_arg}
}
# indent free
str=$(concat "hello1\n" \
"hello2\n" \
hello3)
printf $str"\n"
結果:
hello1
hello2
hello3
答え3
@Anonの問題に対する解決策は実際には非常に簡単です。関数メソッドを使用することができ、各行にprintfを使用せずに、複数行のコメントにも単一のまつげ方法が機能します。
解決策:
echo "This is the first line. " \
> "This is second line"
出力:
This is first line. This is second line
このメソッドを使用して、単一のechoコマンドで複数の文字列を連結できます。スラッシュを使用すると、新しい文を追加できる2番目の空白行が作成されます。
答え4
引用符の中に改行を追加できます。つまり、引用符が同じ行で終わる必要がないため、連続した文字がなくても複数行の文字列を持つことができます。この試み:
#! /bin/bash
echo "This text has
several lines.
This is another
sentence."
echo "This line is printed starting from the left margin
and these lines
are indented
by four spaces"
printf "This text contains
not %d, but %d two substitution
fields.\n" 1 2
これは
This text has
several lines.
This is another
sentence.
This line is printed starting from the left margin
and these lines
are indented
by four spaces
This text contains
not 1, but 2 two substitution
fields.