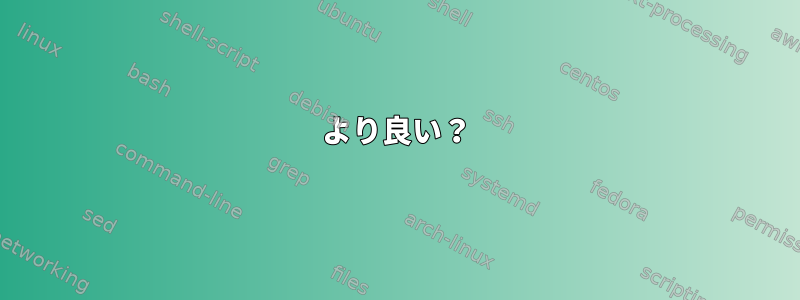
#include<stdio.h>
#include<string.h>
#include<unistd.h>
#include<sys/types.h>
#include<sys/wait.h>
#include<error.h>
#define size 30
char *get_command(int argc, char *argv[]);
int my_system(const char *command);
int my_system(const char *command)
{
int ret = 0;
ret = execl("/bin/sh", "sh", "-c", command, (char *)NULL);
if (ret == -1)
error(1, 0, "error occcured in the execl() system call\n");
return 0;
}
char *get_command(int argc, char **argv)
{
int i = 0;
static char command[size];
strcpy(command, argv[1]);
for (i = 2; i < argc; i++) {
strcat(command, " ");
strcat(command, argv[i]);
}
return command;
}
int main(int argc, char *argv[])
{
pid_t pid;
pid_t ret;
int ret_system;
int i = 0;
int wstatus;
char *command;
if (argc < 2)
error(1, 0, "Too few arguments\n");
printf("The pid of the parent-process is :%d\n", getpid());
pid = fork();
if (pid == -1) {
error(1, 0, "error in creating the sub-process\n");
} else if (pid == 0) {
printf("The pid of the child- process is :%d\n", getpid());
command = get_command(argc, argv);
ret_system = my_system(command);
} else {
ret = waitpid(-1, &wstatus, 0);
printf("The pid of the child that has terminated is %d and the status of exit is %d\n", ret, wstatus);
}
return 0;
}
execコマンドの代わりにexecシェルを使用せずにfork()をmy_system関数に移動しようとしていますが、それは難しくありません。私を助けてください。私は初心者です。ありがとうございます。
int my_system(const char *command)
{
pid_t pid;
int wstatus = 0;
int ret = 0;
if (command == NULL)
return 1;
pid = fork();
if (pid == -1)
return -1;
else if (pid == 0) {
execle("/bin/sh", "sh", "-c",command, (char *)NULL);
} else {
ret = waitpid(-1, &wstatus, 0);
}
return wstatus;
}
char *get_command(int argc, char **argv)
{
int i = 0;
static char command[size];
if (argc == 1)
return NULL;
strcpy(command, argv[1]);
for (i = 2; i < argc; i++) {
strcat(command, " ");
strcat(command, argv[i]);
}
return command;
}
int main(int argc, char *argv[])
{
int ret;
char *command;
command = get_command(argc, argv);
ret = my_system(command);
if (ret == 1)
printf("Command is NULL, shell is available\n");
else if (ret == -1)
printf("Child process could not be created\n");
else
printf("The status is :%d\n", ret);
return 0;
}
答え1
組み込みシステムコールと同様に、システムコールを行うにはそのコールに入る必要がありますfork
。
get_command
エラーがあります。スタック変数へのポインタを返します。その動作は定義されていません(しばらくの間動作した後に停止することがあります)。
戻り値を確認する必要はありませんexec
。返された場合はエラーがあります。したがって、ret_system変数は0のみを受け取ります。
また、シェルは必要ありません(使用したくない場合:ワイルドカード、変数の拡張。そうしません)。
より良い?
system
移植可能です(MS-Windowsおよびその他のサブオペレーティングシステム)。fork
そしてexec
さらに強力になりました。たとえば、パイプを設定できます。- ほとんどの目的に適した抽象化を提供する他の高度なライブラリがあります。
- 高レベルのライブラリを使用する(または直接抽象化する)方が良い場合でも、
fork
andを使用することは学習に最適です。exec