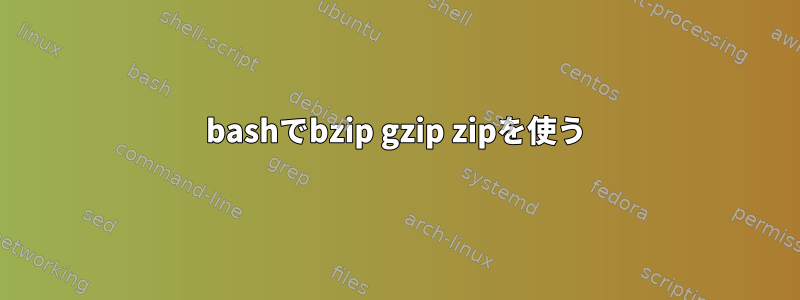
#!/bin/bash
# check if the user put a file name
if [ $# -gt 0 ]; then
# check if the file is exist in the current directory
if [ -f "$1" ]; then
# check if the file is readable in the current directory
if [ -r "$1" ]; then
echo "File:$1"
echo "$(wc -c <"$1")"
# Note the following two lines
comp=$(bzip2 -k $1)
echo "$(wc -c <"$comp")"
else
echo "$1 is unreadable"
exit
fi
else
echo "$1 is not exist"
exit
fi
fi
$1
現在私の問題は、bzipでファイルを単一のファイルに圧縮できることですが$1.c.bz2
、圧縮ファイルのサイズをキャプチャするとどうなりますか?私のコードにはそのようなファイルは表示されません。
答え1
私はあなたのためにコードを整理しました:
#!/bin/bash
#check if the user put a file name
if [ $# -gt 0 ]; then
#check if the file is exist in the current directory
if [ -f "$1" ]; then
#check if the file is readable in the current directory
if [ -r "$1" ]; then
echo "File:$1"
echo "$(wc -c <"$1")"
comp=$(bzip2 -k $1)
echo "$(wc -c <"$comp")"
else
echo "$1 is unreadable"
exit
fi
else
echo "$1 is not exist"
exit
fi
fi
fi
最後から2行目の追加を確認してください。
bzip2 -k test.file
11行目は出力が生成されないため意味がありません。したがって、変数はcomp
空です。
簡単な方法は、拡張が何であるかを簡単に知ることである.bz2
ため、次のようにすることができます。
echo "$(wc -c <"${1}.bz2")"
comp
そして変数はまったく使用されません。
答え2
bzip2 -k
何も出力されないので、コマンドの代わりに実行して変数に出力をキャプチャすると、そのcomp
変数は空になります。この空の変数は主な問題です。この方法を使用することは、ファイルサイズをランダムに取得するよりstat
も優れています。これは、ファイルからすべてのデータを読み取る必要がある面倒ではなく、ファイルのメタデータからファイルサイズのみを読み取る方が効率的です。wc -c
stat
また、スクリプトの作成を妨げるファイルの存在と読みやすさの不要なチェックがたくさんあります。これらのテストはbzip2
とにかく行われ、この事実を利用してほとんどのテストを直接テストする必要はありません。
提案:
#!/bin/sh
pathname=$1
if ! bzip2 -k "$pathname"; then
printf 'There were issues compressing "%s"\n' "$pathname"
echo 'See errors from bzip2 above'
exit 1
fi >&2
size=$( stat -c %s "$pathname" )
csize=$( stat -c %s "$pathname.bz2" )
printf '"%s"\t%s --> %s\n' "$pathname" "$size" "$csize"
スクリプトは終了ステータスを使用して、bzip2
ファイル圧縮が成功したかどうかを確認します。失敗した場合は、bzip2
端末にすでに生成された診断メッセージを補完する診断メッセージを印刷します。
-k
圧縮されていないファイルを保存するためにwithを使用しているため、bzip2
ステートメントの後に両方のファイルのサイズを取得できますif
。-k
コマンドから削除するには、もちろんif
ステートメントの前に圧縮されていないファイルのサイズを取得する必要があります。
スクリプトで使用されている方法は、stat
スクリプトがLinuxシステムで実行されていると想定しています。このstat
ユーティリティは非標準であり、他のシステムの他のオプションと一緒に実装されています。たとえば、macOSやOpenBSDstat -f %z
ではstat -c %s
。
テスト:
$ ./script
bzip2: Can't open input file : No such file or directory.
There were issues compressing ""
See errors from bzip2 above
$ ./script nonexisting
bzip2: Can't open input file nonexisting: No such file or directory.
There were issues compressing "nonexisting"
See errors from bzip2 above
$ ./script file
"file" 600 --> 43
$ ./script file
bzip2: Output file file.bz2 already exists.
There were issues compressing "file"
See errors from bzip2 above
$ rm -f file.bz2
$ chmod u-r file
$ ./script file
bzip2: Can't open input file file: Permission denied.
There were issues compressing "file"
See errors from bzip2 above