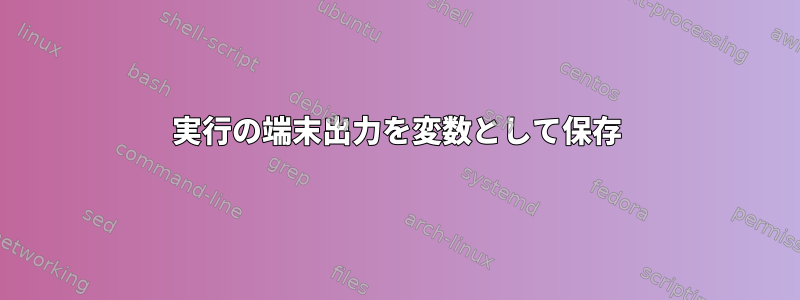
package.jsonを使用してPythonスクリプトを実行していますpyserial
。ボードを使用してモーターの回転を制御し、USBポートを介して接続します。
ボードは、与えられたコマンドに従ってモータを回転するようにプログラムされています。ここにいくつかの例があります。
- 入力:モータ状態確認命令:H010100
出力:
{
.."beambreak": [0,0,0,0,0],
.."prox": [0.003,0.003],
.."T1": [0,0],
.."T2": [0,0],
.."MT": [-1,-1]
.."test_switch": 0,
.}
- 入力:モータ1回転指令:H010101
出力: {"回転":"成功"}
仕事: "while"ループで1分ごとにコマンド(例:H010101)を送信する方法、出力メッセージ(例:{"Rotate":"Successful"})を確認し、出力メッセージの条件に応じて次のコマンドを送信する方法。
質問:コードを実行すると、Linuxターミナル/IDEコンソールに「設定可能」出力メッセージが表示されます。メッセージを変数として保存し、ループ条件に適用する方法がわかりません。つまり、メッセージが同じメッセージであることを確認して1分待ってから、H010101コマンドを再送信しますか?
また、*.logまたは*.txtにファイルを保存しようとしましたが、機能しません。例:
$ python test.py >> *.txt
$ python test.py > *.log
これは私のコードです。
import time
import serial
# configure the serial connections
ser = serial.Serial(
port='/dev/ttyUSB0',
baudrate=115200,
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS
)
while True :
print(' Check Status')
ser.write('H010000\n'.encode())
status_cmd = ser.readline()
print(status_cmd)
if status_cmd === "test_switch: 0 " : # i can't save the message as variable from running terminal
time.sleep(5)
# Command to rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
if reading == {"Drop":"Successful"} : # i can't save the message as variable from running terminal
time.sleep(60)
# rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
答え1
最初にできることは、関数をメソッドにカプセル化することです(または必要に応じてクラスを使用すること)。
状態確認
def check_status(ser):
print('Check Status')
ser.write('H010000\n'.encode())
current_status = ser.readline().decode('utf-8')
return current_status
回転モーター
def rotate_motor(ser):
print('Rotating ..')
ser.write('H010101\n'.encode())
rotation_status = ser.readline().decode('utf-8')
return rotation_status
また、応答をdictにロードするにはjsonを取得する必要があります。
例えば
>>> import json
>>> rotation_status='{"Drop":"Successful"}'
>>> json.loads(rotation_status)
{'Drop': 'Successful'}
これで、これらのコードスニペットが用意されているので、それを呼び出して結果に基づいてジョブを継続的に実行できます。
while True:
status = json.loads(check_status(ser))
if status['test_switch'] == 0:
time.sleep(5)
is_rotated = json.loads(rotate_motor(ser))
if is_rotated['Drop'] == 'Successful':
time.sleep(60)
else:
print('Something went wrong')
raise Exception(is_rotated)