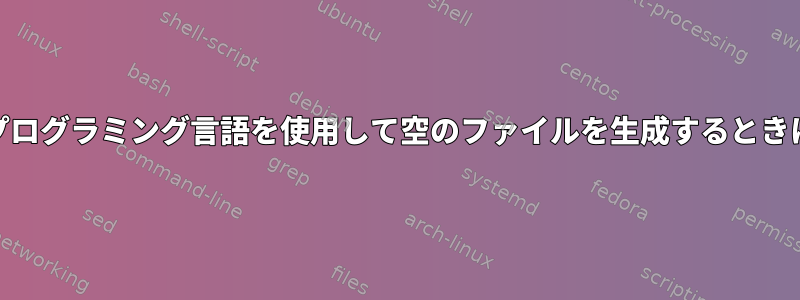
私は最近UNIX環境でプログラミングを始めました。このコマンドを使用して、端末で指定された名前とサイズで空のファイルを生成するプログラムを作成する必要があります。
gcc foo.c -o foo.o
./foo.o result.txt 1000
ここで、result.txtは新しく作成されたファイルの名前を表し、1000はファイルサイズ(バイト単位)を表します。
私は確信しています探す関数はファイルオフセットを移動しますが、問題はプログラムを実行するたびに指定された名前のファイルを生成しますが、ファイルサイズが次のようになることです。0。
これは私のアプレットのコードです。
#include <unistd.h>
#include <stdio.h>
#include <fcntl.h>
#include <ctype.h>
#include <sys/types.h>
#include <sys/param.h>
#include <sys/stat.h>
int main(int argc, char **argv)
{
int fd;
char *file_name;
off_t bytes;
mode_t mode;
if (argc < 3)
{
perror("There is not enough command-line arguments.");
//return 1;
}
file_name = argv[1];
bytes = atoi(argv[2]);
mode = S_IWUSR | S_IWGRP | S_IWOTH;
if ((fd = creat(file_name, mode)) < 0)
{
perror("File creation error.");
//return 1;
}
if (lseek(fd, bytes, SEEK_SET) == -1)
{
perror("Lseek function error.");
//return 1;
}
close(fd);
return 0;
}
答え1
ファイルの終わりを見ている場合は、その場所に少なくとも1バイトを書き込む必要があります。
write(fd, "", 1);
オペレーティングシステムにスペースをゼロで埋めるようにします。
したがって、特定のサイズの1000の空のファイルを生成するには、lseek
次の手順を実行します。
lseek(fd, 999, SEEK_SET); //<- err check
write(fd, "", 1); //<- err check
ftruncate
おそらくより良いだろうし、簡単にまれなファイルを生成するようです。
ftruncate(fd, 1000);
答え2
ファイルに何も書きませんでした。
ファイルを開き、ファイル記述子を移動して閉じます。
lseek のマニュアルページから
lseek() 関数は、ファイル記述子 fildes のオフセットを命令に従って引数オフセットに再配置します。
答え3
マンページから -
The lseek() function allows the file offset to be set beyond the end of the file (but this does not change the size of the file). If
data is later written at this point, subsequent reads of the data in the gap (a "hole") return null bytes ('\0') until data is actually written into the gap.
呼び出しを使用してtruncate/ftruncate
ファイルサイズを設定します。